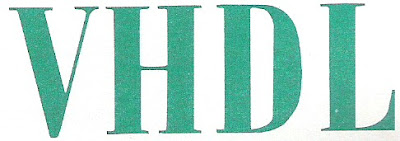
- Introduction to VHDL
- VHDL stands for Very High Speed Integrated Circuit Hardware Description Language. The language was developed in the early 1980s.
- Initially funded by the US Department of Defence.
- It was defined as an IEEE Standard-1076 in 1987.
- Originally meant for design standardization, documentation, simulation and ease of maintenance.
- VHDL, first HDL language to be standardized by IEEE.
- An updated standard IEEE-1164 was later added to introduce multi-value logic system.
- VHDL, intended for simulation as well as circuit synthesis; not all constructs defined are synthesizable.
- A updated version was defined during 1993, to add some new capabilities, remove some ambiguities, while remaining upwardly compatible.
- In 1996, IEEE 1076.3 became a VHDL synthesis standard.
Why VHDL?
Public availability - VHDL is an IEEE standard. Large designs can be modeled using VHDL and there are no limitations imposed on the size of the design.Design methodology - VHDL supports different design methodologies like top down and library based. It supports design technologies like synchronous and asynchronous.Digital Modeling Techniques – Modeling techniques like finite state machine descriptions, algorithmic descriptions, and Boolean equations can be modeled.Technology and process independence - VHDL does not have an understanding of particular target technologies or processes. Coding using VHDL is basically independent of targets.Target independence - Design description can be targeted to a PLD, FPGA or an ASIC.Wide range of descriptive capability - VHDL supports different descriptive levels like behavioral description to structural description.Reduces Time to Market - By using VHDL the ‘Time to Market’ for large and small designs has been reduced tremendously.Design exchange - VHDL is a standard and VHDL models are guaranteed to run in any system that confirms to that standard.Large scale design - When large designs being executed by multi person teams, elements of VHDL like libraries, packages, configuration etc. support design sharing, design management.Design reuse and supports testing - The language also supports design reuse by enabling the designer to use already designed parts and can be easily tested using test benches.
Primary Design units in VHDL
Configuration - Binds an entity to an architecture when there are multiple architectures for a single entity.Package - Contains frequently used declarations, constants, functions, procedures, user defined data types and components.Library - Consists of all compiled design units like entities, architectures, packages and configurations.
VHDL Code Structure
Library – A collection of commonly used pieces of code. Placing them in the code allows a design to be reused.
Parts of a library
Entity declaration
- Entity defines a new component, its IO connections and related declarations.
- It can be used as a component in any other entity after being compiled into a library.
- Any data type used in an entity must be previously declared in a standard or user defined package.
_____Syntax_____________
entity entity_name is
port port_name : port direction data_type
end entity_name ;
_____example______________
entity my_and is
port ( a : in std_logic; -- Input Port
b : in std_logic; -- Input Port
c : out std_logic); -- Output Port
end my_and;
Entity port is a signal with a specified data flow direction, which provides an interconnection between component and its environment.
The following flow directions are allowed
IN - input. Value can be read but not assignedOUT - output. Value can be assigned but not readINOUT - bi-directional value can be read and assignedBUFFER - out port with internal read capability. Its anoutput port but not a bi-directional port.
Generics - Generics provide means of passing parameters to an entity from outside or during component instantiation.
Allows the same entity/architecture to be used by different designs by changing the generic value.
e.g. (generic bus_width : integer := 8)
___________example______________
entity ex is
generic ( n : integer := 32);
port ( a : in std_logic_vector( n-1 downto 0);
b : out std_logic);
end ex;
Architecture
- Architecture specifies the behavior, function, interconnection and the relationship between the inputs and outputs of an entity.
- An entity can have more than one architecture.
- When multiple architectures are defined, then each is bound to an entity using configuration statement.
- The netlist generated depends upon the way in which codes are written inside the architecture.
- The codes can be written in concurrent and in sequential manner.
- There can be no architecture without an entity.
- Architectures can have various levels of abstraction and implementations.
- It facilitates faster design, better understanding, better performance and lesser complexity.
- It has two parts; the declarative part (optional) and code part (from begin onwards).
- The signals and constants required are declared in the optional part. Any signal needed should be declared before the code begins.
________Syntax______________
architecture architecture_name of entity_name is
signal declarations;
component declarations;
begin
statement1;
statement2;
…………..
…………..
end architecture_name;
VHDL Architectures classification
Behavioral - Defines a sequentially described functioning of the design.Structural - Defines interconnections between previously defined components.Dataflow - Primarily the flow of data through the entity is expressed using concurrent signal assignment statements.
Mixed style of modeling
Possible to mix all the three modeling styles - we could use component instantiation statements (represent structure), concurrent assignment statements (represent dataflow) and process statements (represents behavior).
Concurrent assignments will give combinational logic, whereas behavioral modeling generates sequential modules.
________Behavioral Style__________
entity my_and is
port (a : in std_logic;
b : in std_logic;
c: out std_logic);
end my_and;
architecture my_and_beh of my_and is
begin
process (a,b)
begin
if (a=‘1’ and b=‘1’) then
c<=‘1’;
else
c<=‘0’;
end if;
end process;
end my_and_beh;
________Structural Style___________
entity my_and is
port ( a : in std_logic;
b : in std_logic;
c: out std_logic);
end my_and;
architecture my_and_struct of my_and is
component AND2
port ( x: in std_logic;
y: in std_logic;
z: out std_logic);
end component;
begin
u0:AND2 port map (x => a, y => b, z=> c);
end my_and_struct;
________Data flow Style______________
entity my_and is
port ( a : in std_logic;
b : in std_logic;
c: out std_logic);
end my_and;
architecture my_and_data of my_and is
begin
c <= a and b;
end my_and_data;
Configuration
- Configuration is used to select one of the possibly many architecture bodies that an entity may have.
- The synthesizer ignores configuration.
- Configuration saves re-compile time when some components need substitution in a large design.
______syntax_____________________
configuration configuration_name of entity_name is
for architecture_name is
for architecture_name
end for;
end configuration_name;
__________Example__________________
configuration THREE of FULLADDER isfor STRUCTURALfor INST_HA1, INST_HA2: HAuse entity WORK.HALFADDER(CONCURRENT);end for;for INST_XOR: XORuse entity WORK.XOR2D1(CONCURRENT);end for;end for;end THREE;
___________Program Explanation____
The first and second line contain the configuration name and the binding of the entity and architecture.
Since the architecture STRUCTURAL is a structural description, the components HA and XOR have to be configured. This is done by the two inner for-loops. The first loop specifies that for the two instances INST_HA1 and INST_HA2 of the component HA an entity HALFADDER with its architecture CONCURRENT has to be used.
Library
- Library is a collection of compiled VHDL design units.
- Library promotes sharing of compiled design and hides the source code from the users.
- VHDL does not support hierarchical (nested) libraries.
- WORK and STD are the default libraries provided by the language. They need not be explicitly declared.
- All the compiled designs are put into WORK by default unless specified.
- Commonly used functions, procedures and user data types can be compiled into a user defined library for use in all designs.
- Before accessing any units in a library it needs to be declared. STD and WORK need not to be declared.
____Library declaration Syntax________
library library_name;e.g. library ieee;
The “USE” statement can access components declared inside a library.
use library_name.package_name.item_name ;use library_name.item_name
______example______________
library IEEE ;use IEEE.std_logic_1164.all ;use IEEE.std_logic_1164_unsigned.all ;library my_library ;use my_library.my_package.all ;
Package
- A package is a collection of commonly used subprograms, data types and constants.
- Package promotes code reuse.
- STANDARD and TEXTIO are provided in the STD library that defines useful data types and utilities.
- ‘USE’ statement is used to access components inside a library.
Package consists of two parts:-.
- Package Header - This defines the contents of a package that is made visible after the statement. “use library.package_name.all”.
- Package body - This provides the implementation details of sub programs. Items declared in the body are not visible to the user of the package.
_____Syntax__________________
package package_name isdeclarations ;end package_name ;package body package_name isdeclarations ;sub program body ;end package_name ;
______Example : A simple package___________
library ieee;use ieee.std_logic_1164.all;package my_package istype state is (st1 st2, st3, st4);type color is (red, green, blue);constant vec : std_logic_vector(7 downto 0) := “11110000”;end my_package;
______Example : Package with a function______
package my_package istype state is (st1 st2, st3, st4);constant vec : std_logic_vector(7 downto 0) := “11110000”;function positive_edge (signal s: std_logic) return boolean;end my_package;package body my_package isfunction positive_edge (signal s: std_logic) return boolean isbeginreturn (s’event and s=‘1’);end positive_edge;end my_package;
Basic Language elements in VHDL
- Class
- Objects
- Data types
- Operators
class object data typesignal a : std_logic
Class
There are three different class types.
- Constant.
- Variable.
- Signal.
Constant
- Does not change its value.
- It can be declared in all parts of a program.
- Can be of any type.
- Normally used to make the code more readable.
Example for a constant declaration.
constant a : a_type :=“1010”;
Signals
It connects design entities together and communicates changes in values.
Signals can be declared
In an entity (visible to all the architectures).In an architecture (local to that architecture).In a package (globally available to user of that package).As a parameter of a subprogram (in a function or procedure).
Signals have three properties attached to it.
Type and type attributes.Value.Time (i.e. it has a history).
_________syntax________________
signal signal_name : signal type
e.g. signal a : std_logic;
Signal assignment is done using the assignment symbol ( <= )
e.g. C <= A + B;
- Signal assignment is concurrent outside the process and sequential inside the process.
- Signals take last value assigned to it in a process and are assigned the value only after the completion of the simulation cycle run.
- Signal updates can have a delay specified in their assignment statements.
e.g. clk <= not clk after 10 ns;
Variables
- Variables are identifiers with in a process or a subprogram.
- Variable assignment occurs immediately.
- Variables have only type and value attached to it.
- They don’t have a past history unlike a signal.
- Variables are used for local data storage.
- Variables cannot be used to communicate between two concurrent statements.
_______Syntax_____________________________
variable variable_name : variable_type ;
e.g.
variable I : integer range 0 to 10;
variable count : std_logic_vector(3 downto 0):=“0000”;
Data types
- Each identifier must have a data type which determines the value, that can assume.
- VHDL supports a variety of data types.
- Users can define their own data types and operators in user defined packages.
Character type
The package STANDARD predefines a set of character literals.
Character types are enclosed in single quotes
E.g. ‘a’, ‘A’, ‘1’, ’#’
An array of character type is enclosed in double quotes.
E.g. “warning”, “setup time violation”
Boolean type
The boolean data type can only be true or false.e.g.process(………..)variable john :boolean;beginjohn := aif john then…………………end process;
Integer type
An integer type defines a type whose set of values fall with in specified range.They can be either positive or negativeExamples of type declarationstype index range 0 to 255;type word_length range 0 to 31;
Real type
Defines a value with a real number.They can be either positive or negative.Exponents have to be integers.type data range 0.0 to 15.9;type length range 1.8 to 4.5;
Bit type
Used to represent the value of a digital system.The values allowed are only 0 and 1.Bit vector literals are expressed as a string of bits enclosed in double quotes.e.g. ‘1’ , “1010” , “101100”
Physical types
Used to represent physical quantities like distance, time etc.Time is a predefined physical unit.User can define their own physical types.e.g.: type current range –2147484638 to 2147484637units nA -- base unituA = 1000 nA;mA = 1000 uA;A = 1000 mA;end units;
Std_logic type
std_logic type is a data type defined in the std_logic_1164 package of IEEE library.It defines 9 different states that a digital system can haveIts an enumerated type.The values that an std_logic type can have are‘U’ --- un-initialized‘X’ --- forcing unknown‘0’ --- forcing 0‘1’ --- forcing 1‘Z’ --- high impedance‘W’ --- weak unknown‘L’ --- weak 0‘H’ --- weak 1‘-’ --- don’t care
Enumerated type
An enumeration data type defines a set of user defined values.Boolean, bit, std_logic etc. are some of the predefined enumerated type.If an enumerated object is not intialised then the default value is the left most value of that type.______syntax________________________________type enumerated_type_name is (enumerated literal1,2,3….);e.g. type colour is (red, blue, green);type instruction is (add, sub, mul, div);
Composite type
There are two composite typesArrays --- contains many elements of same type.Records --- contains elements of different type.
Arrays
Arrays can be either uni dimensional or multi dimensional.____Array declaration syntax_________________type array_name is array (index range) of element_type;e.g.type byte is array (7 downto 0) of std_logic;type word is array (integer range <> ) of std_logic;unconstrained array
Multi dimensional array
_______syntax____________________________type array_name is array (index_range, index_range) of element_typeeg:type memory is array (3 downto 0, 7 downto 0) of bit;type byte is array (7 downto 0) of bit ;type memory is array (3 downto 0) of byte;
Record type
An object of a record is composed of elements of same or different types.________example________________________type module isrecordsize :integer range 20 to 200;crit_dly :time;inputs :integer range 0 to 20;output :integer range 0 to 20;end record;
Operators
Logical operators - and, or, nand, nor, xor, xnor, not.
These operators are defined for the type Boolean and bit. The result of a logical operator has the same type as its operands.
Relational operators.
The relational operators defined in VHDL are
= --- equality
/= --- inequality
< --- less than
<= --- less than or equal to
> --- greater than
>= --- greater than or equal to
The result type of all relational operators will be always of the type ‘BOOLEAN’
Shift operators.
The shift operators are
sll --- shift left logical
srl --- shift right logical
sla --- shift left arithmetic
sra --- shift right arithmetic
rol --- rotate left
ror --- rotate right
Arithmetic operators.
+ --- addition
- --- subtraction
& --- concatenation
* --- multiplication
/ --- division
mod --- modulus
rem --- remainder
- The operands of the + and - operators must be of the same numeric type, with the result being same numeric type.
- The result of concatenation will be always an array.
- The * and \ operators are predefined for both operands being of the same integer or real type. The result is also of the same type.
- The rem and mod operators operates on operands of integer types, and the result is also of the same type.
- The result of the rem operator has the sign of its first operand.
- The result of the mod operator has the sign of the second operator.
No comments:
Post a Comment